Lets write Multi Threaded Python Directory Brute forcing script. The Purpose of this post is not to equip you with a tool you can use, But to give you the basic understanding of how you can write your own better-improved tool – How it works.
The Structure of a Directory Brute Forcer
- if URL Exists :
- Open a word list and read all possible directories
- append the possible directory to the URL
- send HTTP request to the URL
- if HTTP response is 200 OK. URL Exists, Directory found.
Lets Code!
Of course we are gonna be using Python requests. Lets get started.
import requests # To send the HTTP Request
import _thread # For Threading
import socket # For testing connection, This is unnecessary since we can test connection using request but whatever.
import random # To shuffle the directory list.
import sys # To read in command line arguments.
We’re gonna be taking in the host name, The number of threads and the word list as command line parameters.
try:
hostname = sys.argv[1]
wordlist = sys.argv[2]
threads = int(sys.argv[3])
except IndexError:
print("usage : ./py <hostname> <wordlist> <number of threads>")
sys.exit(0)
The above snippet will read in command line arguments. Let’s move on to the interesting part.
def brute_force_directory(hostname):
try:
checked = []
with open(wordlist, "r") as wordlist_file:
dirs = wordlist_file.readlines()
random.shuffle(dirs)
for dir in dirs:
if(dir.startswith("#")):
dirs.remove(dir)
else:
dir = dir.strip("\n")
try:
CHECKURL = "http://" + hostname + "/" + dir
if(CHECKURL not in checked):
checkdir = requests.get(CHECKURL).status_code
print("[*] Checking " + CHECKURL)
if(checkdir == 200):
print("[+] Found " + CHECKURL)
else:
print("|_> Code : " + str(checkdir))
checked.append(CHECKURL)
except Exception as e:
print("[-] Error : " + str(e) + " While checking " + CHECKURL)
except KeyboardInterrupt:
sys.exit(0)
This is going to be our main function that is going to do the brute forcing part. We can now run this in how ever many threads we want.
The above snippet opens the word list and reads all of it’s lines. The lines that are going to be the possible directories. It reads them in a list and we shuffle them using random.shuffle
.
We check if the directory name starts with #
. Which can be random comments in the file, So we skip anything that starts with #
using if(dir.startswith("#"))
.
We created a list to store every directory that has been checked to avoid rechecking it. checked=[]
.
Every directory URL checked is added to that list and every directory URL in the list is ignored.
if(CHECKURL not in checked):
<- This only checks the directory url if it’s not in the list.
Now lets move on to the multi threaded part.
scket = socket.socket(socket.AF_INET, socket.SOCK_STREAM, socket.IPPROTO_TCP)
try:
check = scket.connect_ex((hostname, 80))
scket.close()
if(check == 0):
# url exists
print("[+] Starting Directory Brute Force for URL '{}' ...".format(url))
if(threads > 0):
for _ in range(threads):
_thread.start_new_thread(brute_force_directory, (url,))
while(True):
try:
input("[+] Press CTRL+C to quit.")
except KeyboardInterrupt:
sys.exit(0)
else:
print("[-] Cannot reach host {} ...".format(url))
sys.exit(0)
except socket.error:
print("[-] Cannot reach host {} ...".format(url))
sys.exit(0)
except KeyboardInterrupt:
sys.exit(0)
Here we first create a socket and test connection to the host. If it exists, We start the number of supplied threads of the brute forcing function.
This is the basic outline of a Directory Brute Forcing in python. Of course it could be written in other ways as well.
Further improving this code and it should look something like this :
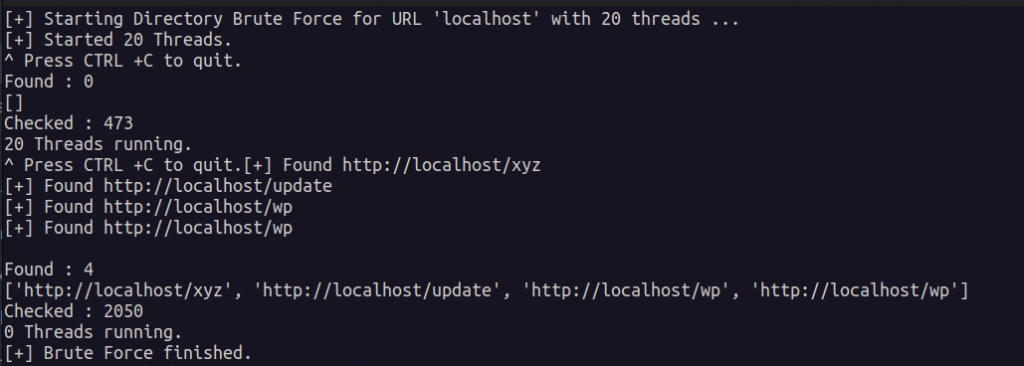
This is Amazing
Hello Fahad,
Please send me an email.
Thanks